1. 데이터를 효율적으로 읽을 때 버퍼링을 사용한다. 이 때 Bufreader의 메서드 read_line()을 쓰면 한줄씩 데이터를 읽어오고 바이트를 반환해준다. (주석 설명 참고)
use std::io::prelude::*;
use std::io::BufReader;
use std::fs::File;
fn main() -> std::io::Result<()>{
let s = File::open("tests/inputs/test.txt")?;
// 버퍼 인스턴스 생성
let mut reader = BufReader::new(s);
// String 타입 인스턴스 생성
let mut line = String::new();
// read_line은 한줄을 읽고 바이트를 반환한다.
let len = reader.read_line(&mut line)?;
println!("바이트 길이 {len}");
Ok(())
}
1-1. test.txt 에는 "한글"이라고 적혀있다 한글 한글자가 3바이트이다.
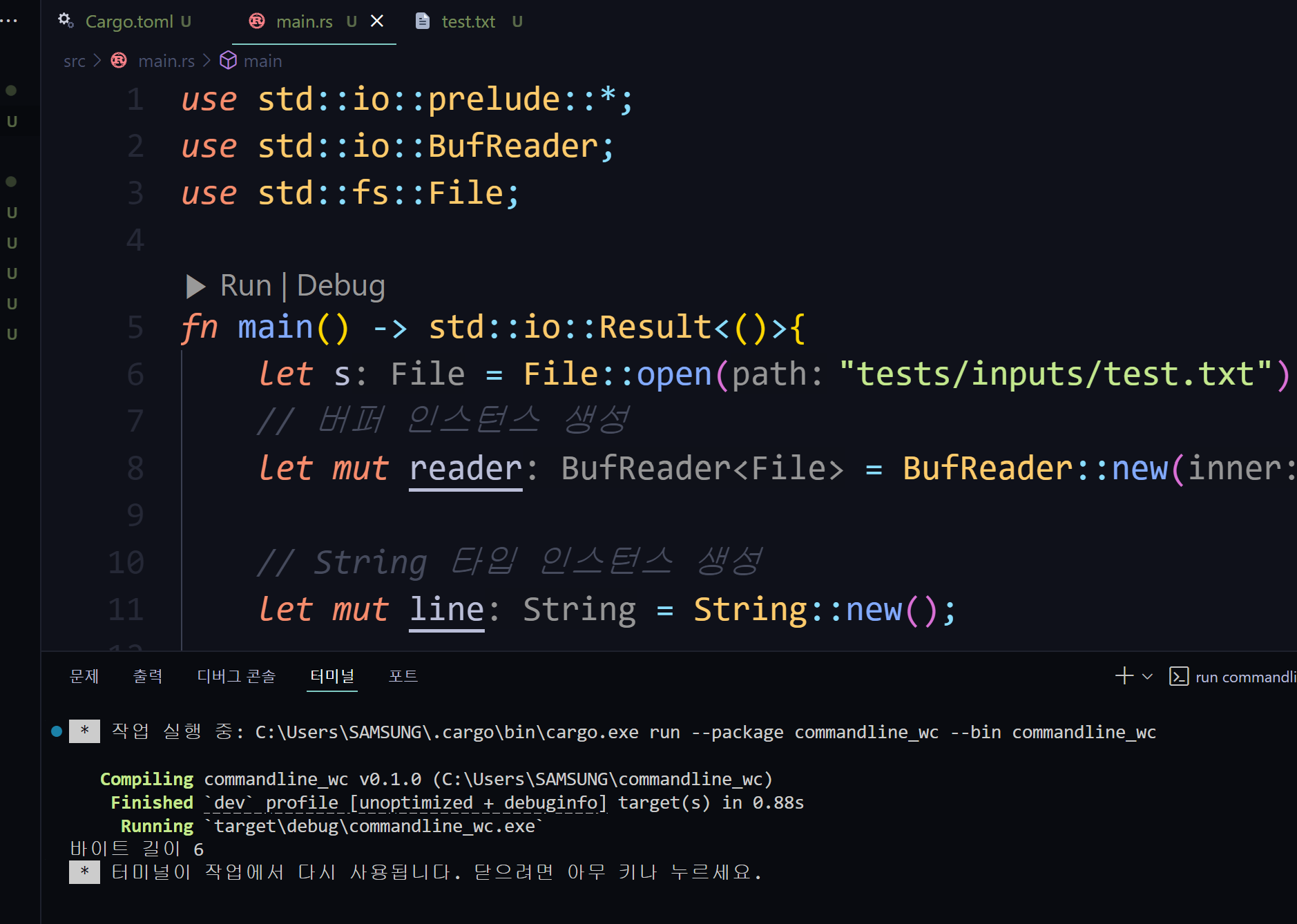
2. Cursor는 AsRef<[u8]>을 구현하는 임의의 인메모리 버퍼를 이용해서 Read나 Write를 따로 구현할 수 있게 해주므로, 이들 버퍼를 실제 I/O를 수행하는 리더나 라이터가 쓰이는 모든 곳에 사용할 수 있다. 쉽게 비유하면 책갈피라고 생각하고 현재 책갈피를 움직이면 다른 페이지로 이동할 수 있듯이, Cursor도 위치를 변경하여 건너뛰어서 데이터를 다른 위치에서 한줄씩 읽거나 쓸 수 있다. 파일을 읽는 테스트를 할 때 유용하다.
use std::io::{self,BufRead};
fn main(){
let mut cursor = io::Cursor::new("한글\n메롱");
let mut buf = String::new();
// 커서를 이용해 한줄씩 읽어 바이트를 반환
// 첫 번째 줄 읽기
let num_bytes = cursor.read_line(&mut buf)
.expect("커서 실패");
assert_eq!(num_bytes,7);
assert_eq!(buf,"한글\n");
buf.clear();
// 두번 째 줄 읽기
let num_bytes = cursor.read_line(&mut buf)
.expect("커서실패");
assert_eq!(num_bytes,6);
assert_eq!(buf, "메롱");
buf.clear();
// End of File (EOF) 마지막 번째 줄 읽기
let num_bytes = cursor.read_line(&mut buf)
.expect("커서실패");
assert_eq!(num_bytes,0);
assert_eq!(buf, "");
}
공식문서를 참고했습니다.
https://doc.rust-lang.org/std/io/struct.BufReader.html
BufReader in std::io - Rust
Seeks relative to the current position. If the new position lies within the buffer, the buffer will not be flushed, allowing for more efficient seeks. This method does not return the location of the underlying reader, so the caller must track this informat
doc.rust-lang.org
https://doc.rust-lang.org/stable/std/io/struct.Cursor.html
Cursor in std::io - Rust
Calls U::from(self). That is, this conversion is whatever the implementation of From for U chooses to do.
doc.rust-lang.org
'Rust' 카테고리의 다른 글
러스트 뮤텍스와 세마포어 (0) | 2024.08.16 |
---|---|
러스트로 리눅스 wc명령어 구현 (0) | 2024.08.14 |
Rust 아토믹(atomic)연산 스레드 (0) | 2024.02.04 |
Rust 스레드 파킹 (0) | 2024.02.04 |
Rust 스레드 조건 변수 대기와 알림 (0) | 2024.02.04 |