Arc<T> 스마트포인터는 참조 카운터를 변경하는 타입이고 병렬처리에서 스레드 간 금액 데이터를 안전하게 공유할 수 있다.
Mutex 스레드를 사용해 스레드를 잠금했다. 잠긴 스레드에 데이터를 엑세스 하고 싶으면 lock메서드를 사용하여 잠금을 풀 수 있다. 그리고 Mutex는 여러스레드가 동시에 데이터에 엑세스 할 수 없다. 예제에서는 금액인출에 대해 트랜잭션 ACID를 구현해 보았다.
use std::sync::{Arc, Mutex};
use std::thread;
use std::time::Duration;
fn alarm(balance: Arc<Mutex<i32>>, amount: i32) {
// Arc<T>를 통해 금액 데이터를 안전하게 공유한다
// lock 메서드를 사용해 데이터 엑세스 허용
let mut balance = balance.lock().unwrap();
println!("{:?}: 인출 시도 중...", thread::current().id());
if *balance >= amount {
// 3초 시간 설정
thread::sleep(Duration::from_millis(3000));
*balance -= amount;
println!("인출 성공! 남은 금액: {balance}");
} else {
println!("금액이 부족합니다.");
}
}
// 스레드 간에 금액들을 공유하고 참조카운터를 변경한다.
fn main() {
//보유 돈: 만원
let balance = Arc::new(Mutex::new(10000));
// 천원 인출
let balance1 = Arc::clone(&balance);
let t1 = thread::spawn(move || alarm(Arc::clone(&balance1), 1000));
// 2만원 인출
let balance2 = Arc::clone(&balance);
let t2 = thread::spawn(move || alarm(Arc::clone(&balance2), 20000));
// 에러처리
t1.join().unwrap();
t2.join().unwrap();
}
결과는 트랜잭션 ACID가 잘 구현되었다.
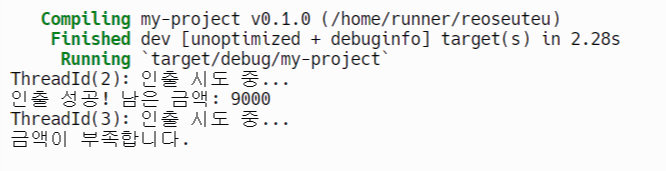
'Rust' 카테고리의 다른 글
Rust 스레드 조건 변수 대기와 알림 (0) | 2024.02.04 |
---|---|
타입 가드 (0) | 2024.01.28 |
자바스크립트 러스트 클로저 비교, 메모리관리 (0) | 2024.01.26 |
Rust Closure (0) | 2024.01.24 |
러스트 impl 트레이트 반환타입 (0) | 2024.01.16 |