인데스란 전체 데이터 조회할때 속도를 높이는 것을 말한다 인덱스 구조는 B-TREE로 되어있고 지금부터 node js를 사용해 실습을 해보겠다.
const MongoClient = require("mongodb").MongoClient;
const url =
"mongodb+srv://id:password@클러스터정보/test?(데이터베이스이름)retryWrites=true&w=majority";
const client = new MongoClient(url, { useNewUrlParser: true });
const express = require("express");
const app = express();
async function main() {
try {
await client.connect();
console.log("MongoDB 접속 성공");
const collection = client.db("test").collection("person");
await collection.insertMany([
{ name: "Hongsun", job: "dev Computer programmer", age: "29" },
{ name: "ongOk", job: "Network administrator ", age: "55" },
{ name: "Suck", job: " Web developer dev", age: "45" },
{ name: "Yeongsu", job: "Network analyst", age: "61" },
{ name: "Lee", job: "Quality assurance dev engineer", age: "33" },
{ name: "Jinsung", job: "PM Network", age: "22" },
{ name: "Jaehyeon", job: "Bacsu", age: "26" },
]);
console.log("문서 추가 완료");
} catch (err) {
console.error(err);
}
}
// mongoDB연결
main();
const collection = client.db("test").collection("person");
app.get("/search", async (req, res) => {
var search = [
{
$search: {
index: "dev2",
text: {
// 쿼리스트링
query: req.query.value,
// 데이터가 저장된 항목들
path: ["name", "job", "age"],
// 유사한 단어를 찾아준다.
fuzzy: {},
},
},
},
];
// 찾은 단어는 배열로 반환해준다.
const documents = await collection.aggregate(search).toArray();
res.json(documents);
});
app.listen(8000, async () => {
console.log("8000포트 실행중");
});
몽고 db를 연결하고 데이터베이스 test안에 있는 컬렉션이름을 person으로 만들었다. aggregate연산자를 사용했는데 그 안에서 또다른 필요한 연산을 할 수 있다. 그다음에는 fuzzy를 활성화 했는데 검색어와 유사한 문자열을 찾을 수 있다. 예시로 dev라는 검색어를 찾을려고하면...
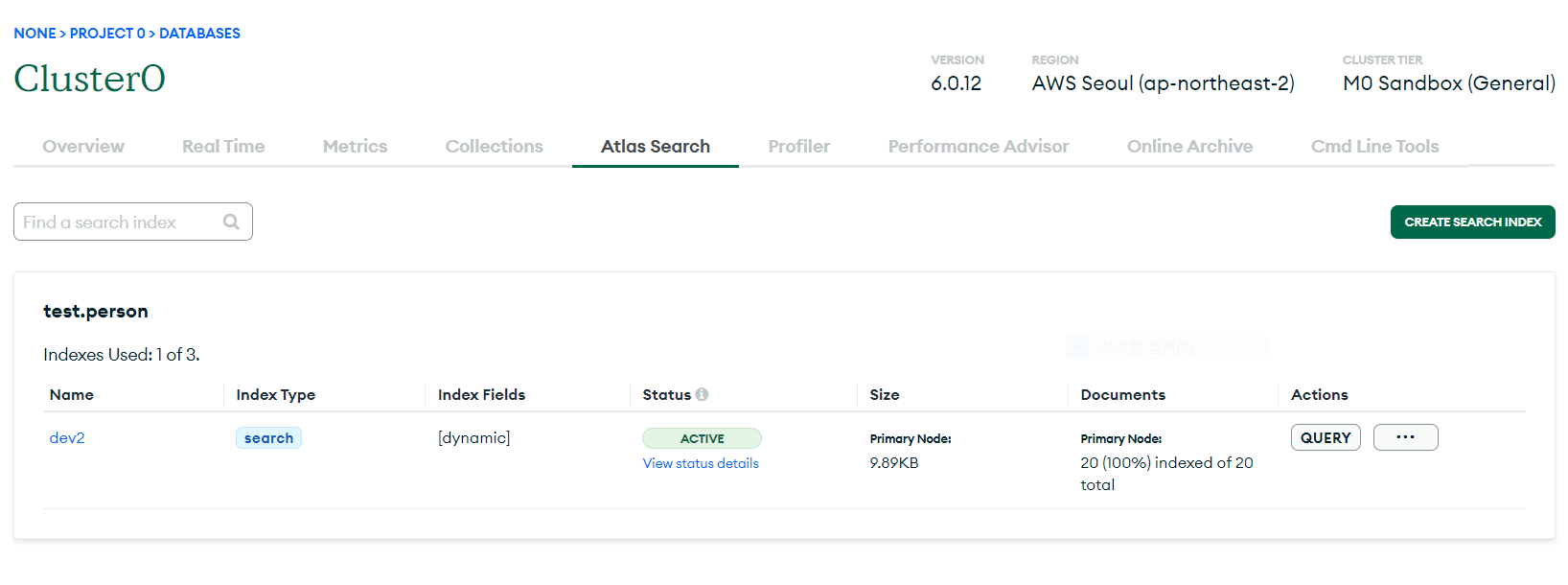
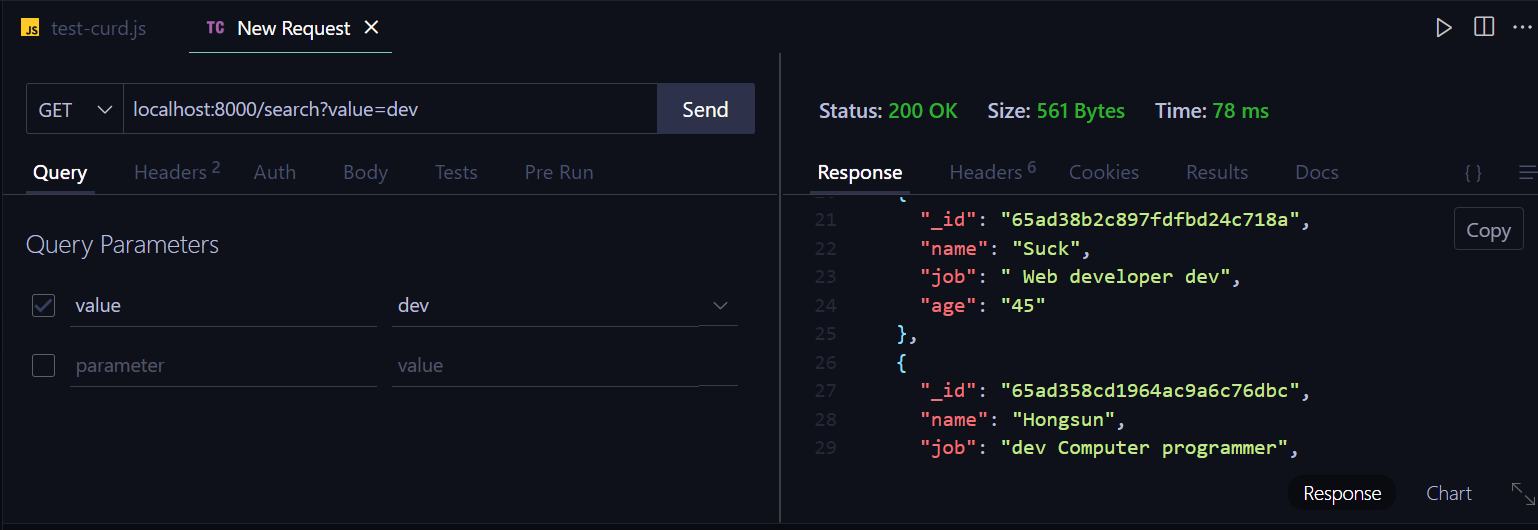
참고자료들
https://www.mongodb.com/docs/manual/indexes/
Indexes — MongoDB Manual
Docs Home → MongoDB Manual Indexes support efficient execution of queries in MongoDB. Without indexes, MongoDB must scan every document in a collection to return query results. If an appropriate index exists for a query, MongoDB uses the index to limit t
www.mongodb.com
https://www.mongodb.com/docs/atlas/atlas-search/text/
text — MongoDB Atlas
Docs Home → MongoDB Atlas The text operator performs a full-text search using the analyzer that you specify in the index configuration. If you omit an analyzer, the text operator uses the default standard analyzer.text has the following syntax:{ $search:
www.mongodb.com
'portfolio' 카테고리의 다른 글
장고(DRF) + 리액트 REACT.JS 포트폴리오 1 (0) | 2024.02.27 |
---|---|
python GPT3.5 turbo모델을 사용하여 데이터베이스 객체 조회하기 (0) | 2024.02.27 |
웹 보안 HTTPS 설정 (0) | 2024.02.24 |
리눅스 grep 명령어 , 파이프 라인 활용 (0) | 2024.01.29 |
Docker(도커) 및 Docker로 Apache서버 띄우기 실습 (0) | 2024.01.10 |